Free Transactional Email API Designed for Fast and Reliably Delivery
AhaSend is built for delivering transactional emails, fast.
Send, receive, and track emails with AhaSend's free email API.
Integrate in minutes
Our APIs come with OpenAPI specs for easy and fast integration
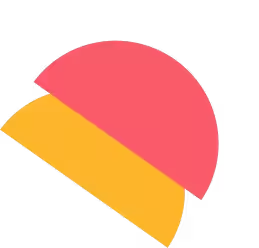
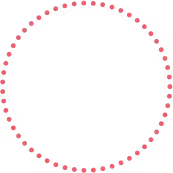

curl https://api.ahasend.com/v1/email/send
-X POST
-H 'Content-Type: application/json'
-H 'X-Api-Key: YOUR-API-KEY'
-d '{
"from": {
"name": "My awesome startup"
"email": "[email protected]"
},
"recipients": [
{
"name": "Someone",
"email": "[email protected]"
}
],
"content": {
"subject": "Sample email",
"text_body": "Plain text body",
"html_body": "<p>This is the <b>HTML</b> body</p>",
}
}'
Install dependencies
$ npm i axios
const axios = require('axios');
const email = {
from: {
name: 'My awesome startup',
email: '[email protected]',
},
recipients: [
{
name: 'Someone',
email: '[email protected]',
}
],
content: {
subject: 'Sample email',
text_body: 'Plain text body',
html_body: '<p>This is the <b>HTML</b> body</p>',
},
};
axios.post('https://api.ahasend.com/v1/email/send', email, {
headers: {
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR-API-KEY',
}
}).then((response) => {
console.log(response);
});
Install dependencies
$ go get gopkg.in/gomail.v2
email := gomail.NewMessage()
email.SetAddressHeader("From", "[email protected]", "My awesome startup")
email.SetAddressHeader("To", "[email protected]", "Someone")
email.SetHeader("Subject", "Sample email")
email.SetBody("text/plain", "Plain text body")
email.SetBody("text/html", "<p>This is the <b>HTML</b> body</p>")
// Port can be 25, 2525, or 587
d := gomail.NewDialer("send.ahasend.com", 587, "YOUR_SMTP_USERNAME", "YOUR_SMTP_PASSWORD")
if err := d.DialAndSend(msg); err != nil {
log.Fatal(err)
}
Install dependencies
$ gem install httparty
require 'httparty'
class AhaSend
include HTTParty
base_uri 'https://send.ahasend.com'
headers 'X-Api-Key' => ENV['AHASEND_API_KEY'], 'Content-Type' => 'application/json'
def initialize(from_name:, from_email:)
@from_name = from_name
@from_email = from_email
end
def send_email(subject:, html_body:, recipient_email:, recipient_name: nil, text_body: nil)
email = {
from: { name: @from_name, email: @from_email },
recipients: [{ name: recipient_name, email: recipient_email }],
content: { subject:, html_body:, text_body: },
}
self.class.post('/v1/email/send', body: email)
end
end
ahasend = AhaSend.new(from_name: 'My awesome startup', from_email: '[email protected]')
ahasend.send_email(
subject: 'Sample email',
html_body: '<p>This is the <b>HTML</b> body</p>',
text_body: 'Plain text body',
recipient_name: 'Someone',
recipient_email: '[email protected]'
)
Install dependencies
$ python -m pip install requests
import requests
email = {
'from': {
'name': 'My awesome startup',
'email': '[email protected]',
},
'recipients': [
{
'name': 'Someone',
'email': '[email protected]',
}
],
'content': {
'subject': 'Sample email',
'text_body': 'Plain text body',
'html_body': '<p>This is the <b>HTML</b> body</p>',
},
}
headers = {
'X-Api-Key': 'YOUR_API_KEY',
'Content-Type': 'application/json'
}
r = requests.post('https://api.ahasend.com/v1/email/send', json=email, headers=headers)
print(r.json())
$email = [
'from' => [
'name' => 'My awesome startup',
'email' => '[email protected]',
],
'recipients' => [
[
name: 'Someone',
email: '[email protected]',
]
],
'content' => [
'subject' => 'Sample email',
'text_body' => 'Plain text body',
'html_body' => '<p>This is the <b>HTML</b> body</p>',
],
];
$options = array(
'http' => array(
'header' => "Content-type: application/json\r\nX-Api-Key: YOUR-API-KEY",
'method' => 'POST',
'content' => json_encode($email)
)
);
$context = stream_context_create($options);
$resp = file_get_contents('https://api.ahasend.com/v1/email/send', FALSE, $context);
var_export($resp, TRUE);
Late delivery is no delivery
When it comes to transactional emails like OTPs and Confirmation emails, delivery speed is everything. AhaSend is built for transactional emails and fast delivery. We consistently deliver emails to Gmail in under 1 seconds, and to other mailbox providers in less than 5 seconds.
Marketing emails are slow and cause delivery delays. We only send transactional emails to ensure high delivery speeds and impeccebale deliverability.
Get your emails to the inbox when it matters.
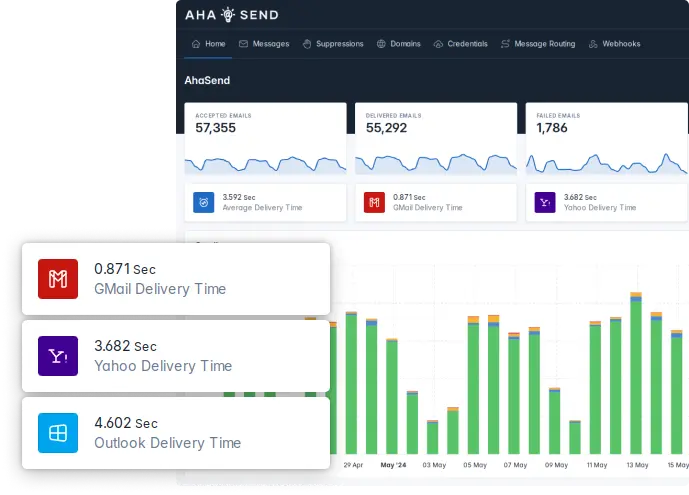
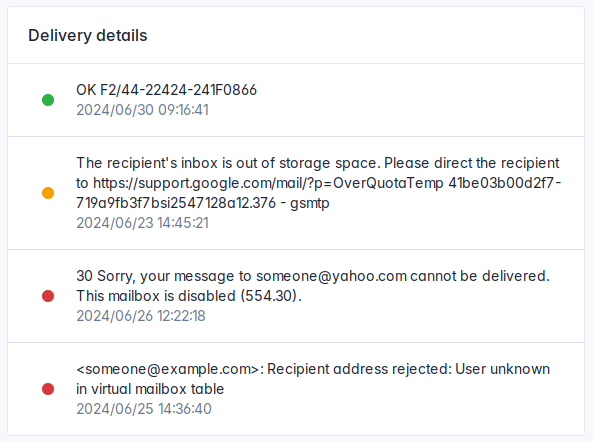
Get access to raw delivery logs
We respect your intellect and don't dumb down or hide delivery logs!
Get access to raw delivery logs and gain visibility on the lowest level of email delivery details.
Quickly debug email delivery and deliverability issues with structured, searchable and raw SMTP response logs.
Webhook Events for Your Emails
Build deep emails integrations using webhooks to receive real-time notifications on
- Email delivery events
- Suppression list events
- Tracking events
- Account related events (such as DNS misconfiguration)
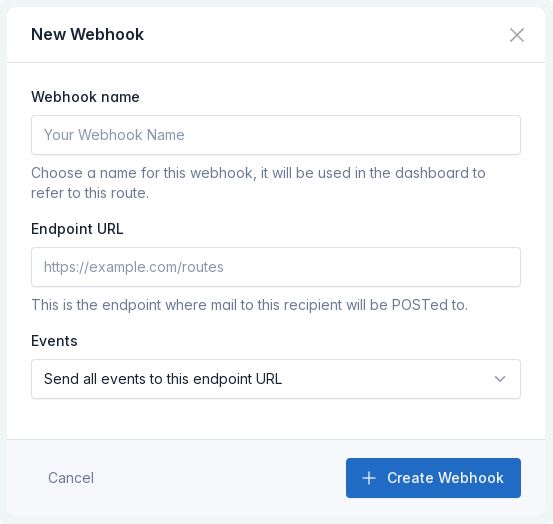
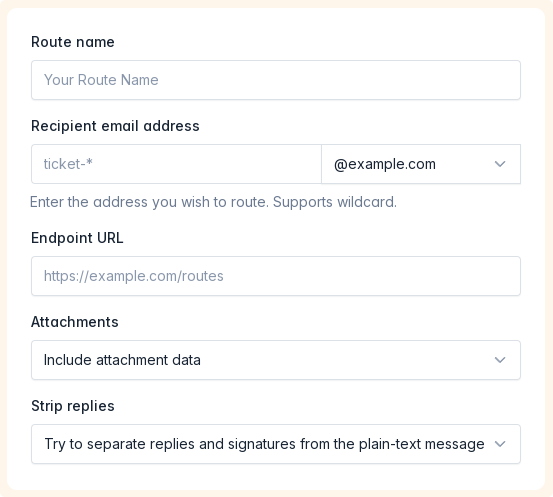
Route your inbound emails
Easily route inbound email to HTTP endpoints. AhaSend automatically parses inbound emails, removing signatures and quoted replies, and provinding the latest reply as a separate field. You can receive structured JSON or raw email data to your endpoints, allowing you to easily integrate emails with your ticketing, CRM or other systems.
Everything you need, included.
More features, at no additional cost. We don't like hidden fees either :)
SMTP Relay
Send emails from any programming language or software using our SMTP relay servers.
Message Retention Policies
Customize retention policies for your message data and metadata.
Email Archiving
Archive your emails on any s3-compatible block storage service for compliance requirements.
Free Dedicated IPs
Large senders sending over 300k emails per month get dedicated IPs - free of charge!
Start delivering your emails to the inbox now.
Integrate AhaSend email APIs in minutes with a free account. Start delivering emails to your customers with 1,000 free emails per month.